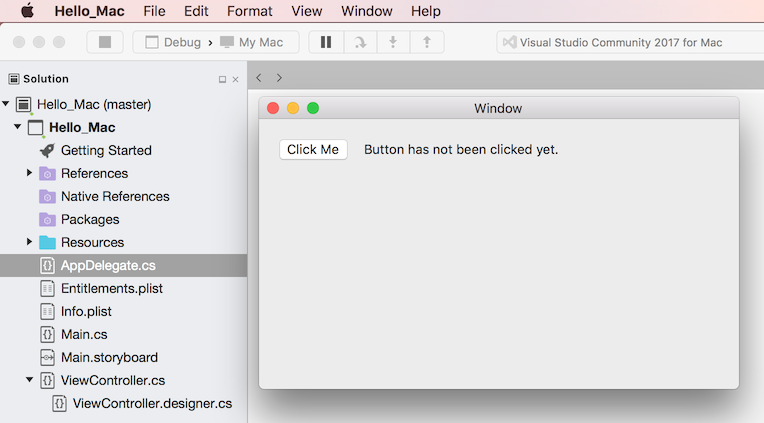
As you can see, in the Color NewC line, I'm using half Alpha (A channel of the pixel), half Red (R channel of the image), half Green (G channel of the image), half Blue (B Channel of the image). Also is better, so you can put the opacity in percetange on the oppacity parameter.
In this step, you make the Show a picture button work as follows:
When a user chooses that button, the app opens an OpenFileDialog box.
If a user opens a picture file, the app shows that picture in the PictureBox.
The IDE has a powerful tool called IntelliSense that helps you write code. As you type code, the IDE opens a box with suggested completions for partial words that you enter.
IntelliSense tries to determine what you want to do next, and automatically jumps to the last item you choose from the list. You can use the up or down arrows to move in the list, or you can keep typing letters to narrow the choices. When you see the choice you want, choose the Tab key to select it. Or, you can ignore the suggestions, if not needed.
To write code for the show a picture button event handler
Go to Windows Forms Designer and double-click the Show a picture button. The IDE immediately goes to the code designer and moves your cursor so it's inside the
showButton_Click()
(alternatively,ShowButton_Click()
) method that you added previously.Type an
i
on the empty line between the two braces{ }
. (In Visual Basic, type on the empty line betweenPrivate Sub..
andEnd Sub
.) An IntelliSense window opens, as shown in the following image.Note
Your code might not display event handlers in 'camelCase' letters.
The IntelliSense window should highlight the word
if
. (If not, enter a lowercasef
, and it will.) Notice how a tooltip box next to the IntelliSense window appears with the description, Code snippet for if statement. (In Visual Basic, the tooltip also states that this is a snippet, but with slightly different wording.) You want to use that snippet, so choose the Tab key to insertif
into your code. Then choose the Tab key again to use theif
snippet. (If you chose somewhere else and your IntelliSense window disappeared, backspace over thei
and retype it, and the IntelliSense window opens again.)
Use IntelliSense to enter more code
Next, you use IntelliSense to enter more code to open an Open File dialog box. If the user chose the OK button, the PictureBox loads the file that the user selected. The following steps show how to enter the code, and although there are many steps, it's just a few keystrokes:
Start with the selected text true in the snippet. Type
op
to overwrite it. (In Visual Basic, you start with an initial cap, so typeOp
.)The IntelliSense window opens and displays openFileDialog1. Choose the Tab key to select it. (In Visual Basic, it starts with an initial cap, so you see OpenFileDialog1. Ensure that OpenFileDialog1 is selected.)
To learn more about
OpenFileDialog
, see OpenFileDialog.Type a period (
.
) (Many programmers call this a dot.) Because you typed a dot right after openFileDialog1, an IntelliSense window opens, filled in with all of the OpenFileDialog component's properties and methods. These are the same properties that appear in the Properties window when you choose it in Windows Forms Designer. You can also choose methods that tell the component to do things (like open a dialog box).Note
The IntelliSense window can show you both properties and methods. To determine what is being shown, look at the icon on the left side of each item in the IntelliSense window. You see an image of a block next to each method, and an image of a wrench (or spanner) next to each property. There's also a lightning bolt icon next to each event.
Here are the icons that appear:Start to type
ShowDialog
(capitalization is unimportant to IntelliSense). TheShowDialog()
method will show the Open File dialog box. After the window has highlighted ShowDialog, choose the Tab key. You can also highlight 'ShowDialog' and choose the F1 key to get help for it.To learn more about the
ShowDialog()
method, see ShowDialog Method.When you use a method on a control or a component (referred to as calling a method), you need to add parentheses. So enter opening and closing parentheses immediately after the 'g' in
ShowDialog
:()
It should now look like 'openFileDialog1.ShowDialog()'.Note
Methods are an important part of any app, and this tutorial has shown several ways to use methods. You can call a component's method to tell it to do something, like how you called the OpenFileDialog component's
ShowDialog()
method. You can create your own methods to make your app do things, like the one you're building now, called theshowButton_Click()
method, which opens a dialog box and a picture when a user chooses a button.For C#, add a space, and then add two equal signs (). For Visual Basic, add a space, and then use a single equal sign (
=
). (C# and Visual Basic use different equality operators.)Add another space. As soon as you do, another IntelliSense window opens. Start to type
DialogResult
and choose the Tab key to add it.Note
When you write code to call a method, sometimes it returns a value. In this case, the OpenFileDialog component's ShowDialog() method returns a DialogResult value. DialogResult is a special value that tells you what happened in a dialog box. An OpenFileDialog component can result in the user choosing OK or Cancel, so its
ShowDialog()
method returns eitherDialogResult.OK
orDialogResult.Cancel
.Type a dot to open the DialogResult value IntelliSense window. Enter the letter
O
and choose the Tab key to insert OK. 888poker for mac download.To learn more about DialogResult, see DialogResult.
Note
The first line of code should be complete. For C#, it should be similar to the following.
if (openFileDialog1.ShowDialog() DialogResult.OK)
For Visual Basic, it should be the following.
If OpenFileDialog1.ShowDialog() = DialogResult.OK Then
Now add one more line of code. You can type it (or copy and paste it), but consider using IntelliSense to add it. The more familiar you are with IntelliSense, the more quickly you can write your own code. Your final
showButton_Click()
method should look similar to the following code.Important
Use the programming language control at the top right of this page to view either the C# code snippet or the Visual Basic code snippet.
Next steps
To go to the next tutorial step, see Step 9: Review, comment, and test your code.
To return to the previous tutorial step, see Step 7: Add dialog components to your form.
See also
- VB.Net Basic Tutorial
- VB.Net Advanced Tutorial
- VB.Net Useful Resources
- Selected Reading
The PictureBox control is used for displaying images on the form. The Image property of the control allows you to set an image both at design time or at run time.
Let's create a picture box by dragging a PictureBox control from the Toolbox and dropping it on the form.
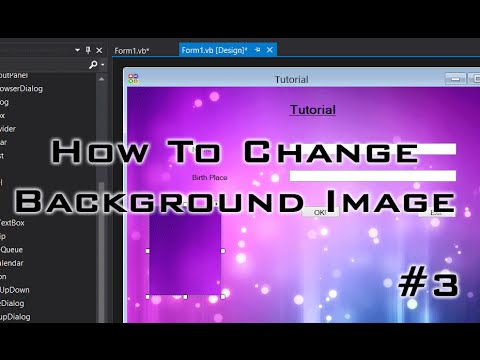
Properties of the PictureBox Control
The following are some of the commonly used properties of the PictureBox control −
Sr.No. | Property & Description |
---|---|
1 | AllowDrop Specifies whether the picture box accepts data that a user drags on it. |
2 | ErrorImage Gets or specifies an image to be displayed when an error occurs during the image-loading process or if the image load is cancelled. |
3 | Image Gets or sets the image that is displayed in the control. |
4 | ImageLocation Gets or sets the path or the URL for the image displayed in the control. |
5 | InitialImage Gets or sets the image displayed in the control when the main image is loaded. |
6 | SizeMode Determines the size of the image to be displayed in the control. This property takes its value from the PictureBoxSizeMode enumeration, which has values −
|
7 | TabIndex Gets or sets the tab index value. |
8 | TabStop Specifies whether the user will be able to focus on the picture box by using the TAB key. |
9 | Text Gets or sets the text for the picture box. |
10 | WaitOnLoad Specifies whether or not an image is loaded synchronously. |
Methods of the PictureBox Control
The following are some of the commonly used methods of the PictureBox control −
Sr.No. | Method Name & Description |
---|---|
1 | CancelAsync Cancels an asynchronous image load. |
2 | Load Displays an image in the picture box |
3 | LoadAsync Loads image asynchronously. |
4 | ToString Returns the string that represents the current picture box. |
Events of the PictureBox Control
The following are some of the commonly used events of the PictureBox control −
Sr.No. | Event & Description |
---|---|
1 | CausesValidationChanged Overrides the Control.CausesValidationChanged property. |
2 | Click Occurs when the control is clicked. |
3 | Enter Overrides the Control.Enter property. |
4 | FontChanged Occurs when the value of the Font property changes. |
5 | ForeColorChanged Occurs when the value of the ForeColor property changes. |
6 | KeyDown Occurs when a key is pressed when the control has focus. |
7 | KeyPress Occurs when a key is pressed when the control has focus. |
8 | KeyUp Occurs when a key is released when the control has focus. |
9 | Leave Occurs when input focus leaves the PictureBox. |
10 | LoadCompleted Occurs when the asynchronous image-load operation is completed, been canceled, or raised an exception. |
11 | LoadProgressChanged Occurs when the progress of an asynchronous image-loading operation has changed. |
12 | Resize Occurs when the control is resized. |
13 | RightToLeftChanged Occurs when the value of the RightToLeft property changes. |
14 | SizeChanged Occurs when the Size property value changes. |
15 | SizeModeChanged Occurs when SizeMode changes. |
16 | TabIndexChanged Occurs when the value of the TabIndex property changes. |
17 | TabStopChanged Occurs when the value of the TabStop property changes. |
18 | TextChanged Occurs when the value of the Text property changes. |
Example
In this example, let us put a picture box and a button control on the form. We set the image property of the picture box to logo.png, as we used before. The Click event of the button named Button1 is coded to stretch the image to a specified size −
Design View −
When the application is executed, it displays −
Clicking on the button results in −